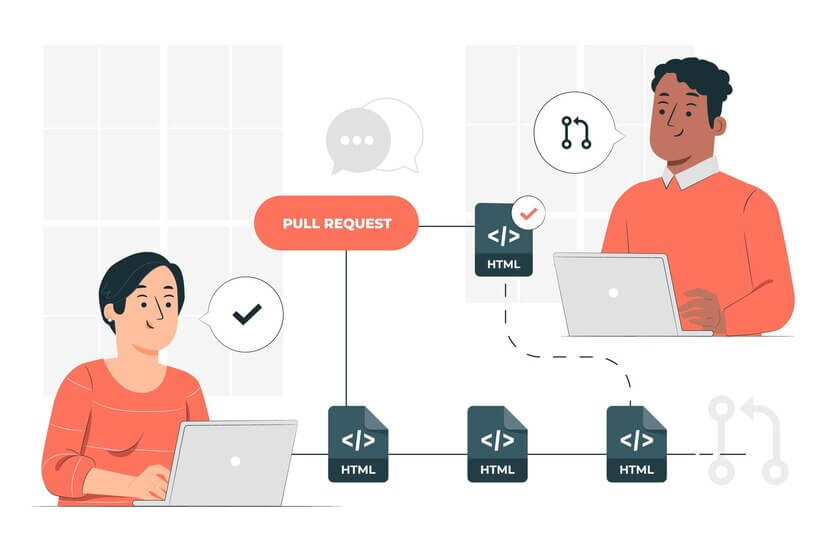
What is Git?
Git is a distributed version control system that enables developers to track changes in their codebase, collaborate with others, and manage project history efficiently. It is a fundamental tool for modern software development.
Git and GitHub
Git is the version control tool, while GitHub is a web-based platform that hosts Git repositories. GitHub facilitates collaboration by providing a central location for code storage, issue tracking, and collaboration among multiple contributors.
Getting Started with Git
Understanding Repositories
A repository is a storage space for a project’s files, often referred to as a codebase. In Git, repositories are hosted on platforms like GitHub. The .git
folder appears when you initialize a Git repository using the command git init
.
Basic Git Commands
Checking Status
Use git status
to view the status of your repository. Untracked files are files that Git is not currently monitoring.
git status
Adding Changes to Staging
To add all files to the staging area, use git add .
. The files in the staging area turn green in the status output.
git add .
Making Commits
After staging changes, commit them using git commit -m "commit message"
. Commits create a snapshot of the project at a specific point in time.
git commit -m "Added new file"
Git Log
Use git log
to see a detailed history of commits. Each commit has a unique identifier, commit message, and other metadata.
git log
Rolling Back Changes
Rollback commits using git reset <commit id>
. To unstage changes, use git restore --stage <file.name>
.
git reset <commit id>
git restore --stage <file.name>
Git Stash
Git stash is used to temporarily save changes that are not ready to be committed. Use git stash
, git stash pop
, and git stash clean
to manage stashed changes.
git stash
git stash pop
git stash clean
Collaborative Development with Git
Working with Remote Repositories
Creating a Repository on GitHub
Create a new repository on GitHub and link it to your local Git repository using git remote add origin <url>
.
git remote add origin <url>
Pushing Changes to GitHub
Use git push origin <branch>
to push changes to the GitHub repository.
git push origin main
Branching and Merging
Create and manage branches using git branch
. Merge branches with git merge <branchname>
.
git branch feature
git merge feature
Git Clone and Git Fork
Use git clone
to copy a repository, and git fork
to create a personal copy of a repository.
git clone <repository-url>
git fork
Pull Requests on GitHub
Create branches for features, make changes, and create pull requests on GitHub to merge changes into the main branch.
git fetch --all --prune
Advanced Git Operations
Git Rebase
Reorganize commit history with git rebase -i <commit id>
. Use pick and squash to combine and reorder commits.
git rebase -i <commit id>
Merge Conflict Resolution
Resolve conflicts with git merge
or git rebase
. Conflicts occur when changes conflict between branches.
git diff <branchname>
Git Ignore
The .gitignore
file specifies files or directories to be ignored by Git, preventing them from being tracked.
Git Hooks
Hooks in the .git/hooks
directory execute custom scripts before or after specific Git actions.
Cherry-Pick
Cherry-pick allows you to apply specific commits from one branch to another.
git cherry-pick <commit-id>
Git Merge vs. Git Rebase
Choose between merging branches with git merge
or reorganizing commit history with git rebase
.
Git Fetch vs. Git Pull
git fetch
retrieves changes from a remote repository, while git pull
fetches changes and merges them into the current branch.
Git Amend
Use git commit --amend
to modify the last commit, combining staged changes with the previous commit.
git commit --amend
Conclusion
Mastering Git is essential for effective collaboration and version control in software development. By understanding the basics and exploring advanced features, DevOps engineers can optimize their workflow and contribute to successful project management.