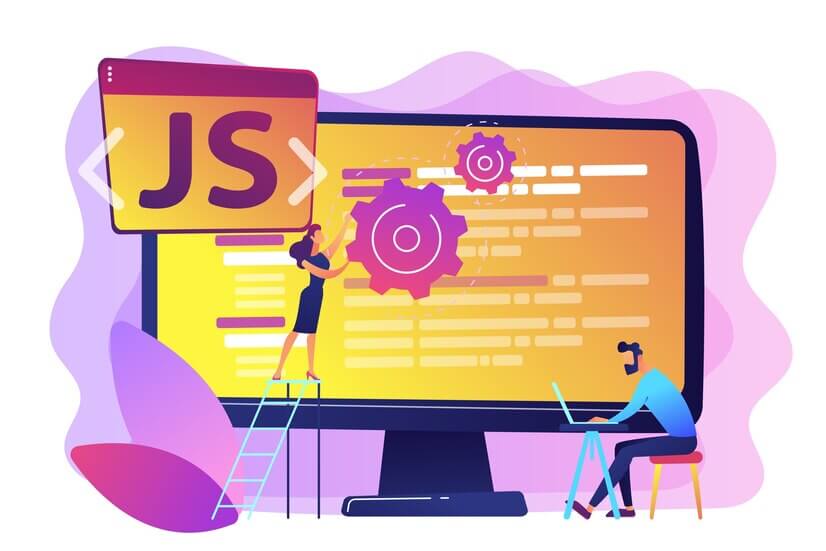
Introduction
JSON (JavaScript Object Notation) is a lightweight data interchange format widely used for transmitting data between a server and a web application. JSON arrays, represented as JSONArray in JavaScript, are a collection of values enclosed in square brackets. Iterating through JSON arrays efficiently is a common requirement in web development, especially when dealing with API responses or data manipulation tasks.
In this article, we’ll explore various methods and techniques to iterate through JSONArray in JavaScript. We’ll cover basic iterations, nested array iterations, and explore some advanced techniques to handle complex JSON structures. Additionally, we’ll provide code examples and explanations to help you understand and implement these concepts effectively in your projects.
Understanding JSONArray in JavaScript
Before diving into iteration techniques, let’s briefly discuss JSONArray in JavaScript. As mentioned earlier, a JSONArray is a collection of values enclosed within square brackets []. These values can be of any data type supported by JSON, such as strings, numbers, booleans, objects, or even nested arrays.
Here’s an example of a simple JSONArray:
var jsonArray = [1, 2, 3, "four", true];
In the above example, jsonArray is a JSONArray containing a mix of numeric, string, and boolean values.
Now, let’s explore various methods to iterate through this JSONArray.
- Using for Loop:
The simplest way to iterate through a JSONArray is by using a for loop. This method is straightforward and works well for linear iterations.
for (var i = 0; i < jsonArray.length; i++) {
console.log(jsonArray[i]);
}
In this example, we use a for loop to iterate through each element of the jsonArray and print its value to the console.
- Using forEach() Method:
The forEach() method is a built-in function available for arrays in JavaScript. It allows you to execute a provided function once for each element in the array.
jsonArray.forEach(function(element) {
console.log(element);
});
In the above code snippet, we use the forEach() method to iterate through each element of the jsonArray and print its value to the console.
- Using for…of Loop:
The for…of loop is a modern iteration construct introduced in ES6 (ECMAScript 2015). It provides a more concise syntax for iterating over iterable objects, including arrays.
for (let element of jsonArray) {
console.log(element);
}
In this example, we use the for…of loop to iterate through each element of the jsonArray and print its value to the console.
Now that we’ve covered basic iteration methods, let’s explore how to handle nested JSON arrays.
Nested JSONArray Iteration
JSONArray can contain nested arrays as elements, forming a multidimensional data structure. Iterating through such nested arrays requires a nested approach. Let’s consider an example of a nested JSONArray:
var nestedJsonArray = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
To iterate through each element of the nestedJsonArray, we’ll use a combination of for loops or forEach() methods for each level of nesting.
nestedJsonArray.forEach(function(innerArray) {
innerArray.forEach(function(element) {
console.log(element);
});
});
In this example, we first iterate through each inner array using the forEach() method, and then within each inner array, we iterate through its elements and print them to the console.
Handling Complex JSON Structures
Sometimes, JSON data can have complex nested structures, including objects within arrays or arrays within objects. Iterating through such structures requires a recursive approach.
Let’s consider an example of a complex JSON structure:
var complexJson = {
"name": "John",
"age": 30,
"contacts": [
{
"type": "email",
"value": "john@example.com"
},
{
"type": "phone",
"value": "123-456-7890"
}
],
"address": {
"city": "New York",
"zip": "10001"
}
};
To iterate through this complex JSON structure, including nested arrays and objects, we’ll define a recursive function.
function iterateJson(jsonObj) {
for (var key in jsonObj) {
if (jsonObj.hasOwnProperty(key)) {
if (Array.isArray(jsonObj[key])) {
jsonObj[key].forEach(function(item) {
iterateJson(item);
});
} else if (typeof jsonObj[key] === 'object') {
iterateJson(jsonObj[key]);
} else {
console.log(key + ': ' + jsonObj[key]);
}
}
}
}
iterateJson(complexJson);
In this recursive function iterateJson(), we iterate through each key-value pair of the JSON object. If the value is an array, we recursively call iterateJson() for each element of the array. If the value is another object, we recursively call iterateJson() for that object. Otherwise, we print the key-value pair to the console.
Conclusion
Iterating through JSON arrays in JavaScript is a fundamental skill for web developers. In this article, we explored various methods and techniques to iterate through JSONArray efficiently. We covered basic iterations using for loops, forEach() method, and modern for…of loop. We also discussed how to handle nested JSONArray and complex JSON structures using recursive approaches.
By mastering these iteration techniques, you’ll be better equipped to manipulate and process JSON data in your web applications effectively.