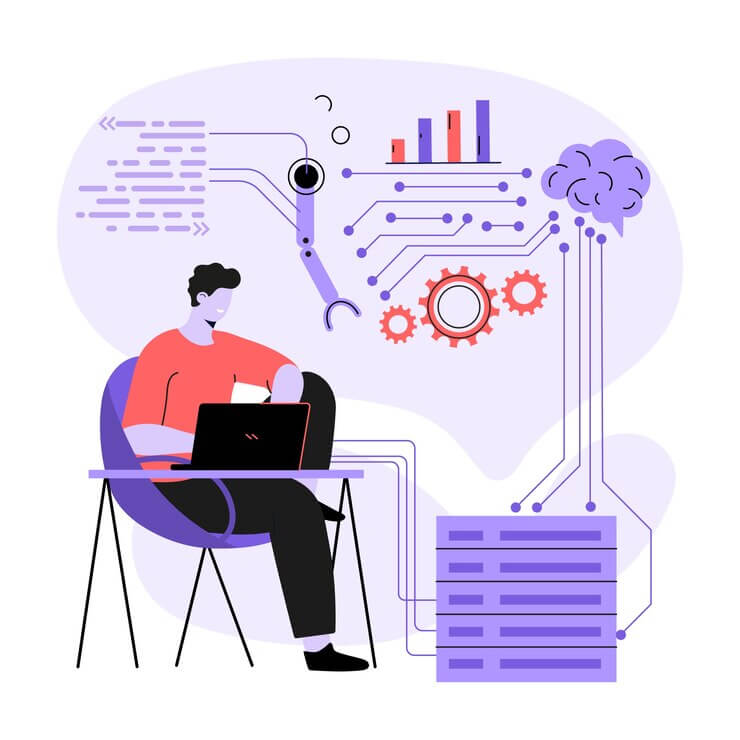
The Banker’s Algorithm is a crucial resource allocation and deadlock avoidance technique in operating systems. It helps ensure that processes in a system can request and release resources in a way that prevents deadlock while maintaining system stability. In this article, we will delve into the intricacies of the Banker’s Algorithm and provide a step-by-step guide on implementing it in C. We’ll also include the necessary code and images to make the learning process easier.
Understanding the Banker’s Algorithm
Before we dive into the code, let’s first understand the core concepts of the Banker’s Algorithm:
- Resources and Processes: In any operating system, resources such as CPU, memory, and I/O devices are limited. Processes running on the system can request and release these resources.
- Resource Allocation: The Banker’s Algorithm allocates resources to processes based on their maximum resource needs, current resource allocations, and available resources. It checks if allocating resources to a process will lead to a safe state, preventing deadlock.
- Safe and Unsafe States: A system is considered to be in a safe state if there is a sequence in which all processes can finish their execution without getting stuck in a deadlock. If no such sequence exists, the system is in an unsafe state.
- Request and Release: Processes can request additional resources and release resources they no longer need. The Banker’s Algorithm ensures that these requests are granted only if they do not lead to an unsafe state.
Now, let’s move on to the step-by-step implementation of the Banker’s Algorithm in C.
Implementing the Banker’s Algorithm in C
Here’s a simplified C program to implement the Banker’s Algorithm:
#include <stdio.h>
int main() {
int processes, resources;
// Input the number of processes and resources
printf("Enter the number of processes: ");
scanf("%d", &processes);
printf("Enter the number of resources: ");
scanf("%d", &resources);
// Define the maximum resource matrix
int max[processes][resources];
printf("Enter the maximum resources for each process:\n");
for (int i = 0; i < processes; i++) {
for (int j = 0; j < resources; j++) {
scanf("%d", &max[i][j]);
}
}
// Define the allocation matrix
int allocation[processes][resources];
printf("Enter the allocated resources for each process:\n");
for (int i = 0; i < processes; i++) {
for (int j = 0; j < resources; j++) {
scanf("%d", &allocation[i][j]);
}
}
// Calculate the need matrix
int need[processes][resources];
for (int i = 0; i < processes; i++) {
for (int j = 0; j < resources; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
// Add your Banker's Algorithm logic here
return 0;
}
Understanding the Code
- We start by inputting the number of processes and resources.
- We then create matrices for maximum resources (
max
), allocated resources (allocation
), and resource needs (need
). - The program prompts the user to input the maximum and allocated resources for each process.
- The need matrix is calculated by subtracting the allocated resources from the maximum resources for each process.
Note: The actual Banker’s Algorithm logic (checking for a safe state and resource allocation) is not included in the provided code. Implementing this logic is the next step in the process.
Frequently asked questions (FAQs) about the Banker’s Algorithm
Q1: What is the primary purpose of the Banker’s Algorithm in operating systems?
A: The Banker’s Algorithm serves two primary purposes in operating systems:
- Resource Allocation: It ensures that resources are allocated to processes in a safe manner, preventing deadlock.
- Deadlock Avoidance: It helps avoid situations where processes are unable to proceed due to a lack of necessary resources, which could lead to a system-wide deadlock.
Q2: What are the key components of the Banker’s Algorithm?
A: The Banker’s Algorithm involves three main data structures:
- Maximum: A matrix that represents the maximum resources each process may request.
- Allocation: A matrix that represents the resources currently allocated to each process.
- Need: A matrix that represents the resources a process still needs to complete its task (Maximum – Allocation).
Q3: How does the Banker’s Algorithm ensure deadlock avoidance?
A: The Banker’s Algorithm ensures deadlock avoidance by only granting resource requests if it can guarantee that the system remains in a safe state after the allocation. It checks if there is a safe sequence of processes that can complete their execution without leading to deadlock. If such a sequence exists, the resource request is granted; otherwise, it is denied.
Q4: What is a safe state in the context of the Banker’s Algorithm?
A: A safe state is a state in which there exists a sequence of processes that can execute to completion without encountering a deadlock. In a safe state, all resource requests can be satisfied, and no process is forced to wait indefinitely.
Q5: How does the Banker’s Algorithm handle resource requests from processes?
A: When a process requests additional resources, the Banker’s Algorithm first checks if the requested resources can be granted without violating the safety conditions. If the resources can be allocated without causing an unsafe state, they are granted; otherwise, the process is forced to wait until it can be safely allocated.
Q6: What are the risks of using the Banker’s Algorithm?
A: While the Banker’s Algorithm is effective at avoiding deadlocks, it has some limitations:
- It assumes that the maximum resource needs of each process are known in advance, which may not always be the case.
- It may lead to resource underutilization, as resources are allocated conservatively to ensure deadlock avoidance.
Q7: Can the Banker’s Algorithm handle dynamic allocation and deallocation of resources?
A: Yes, the Banker’s Algorithm can handle dynamic allocation and deallocation of resources. When a process releases resources, they become available for allocation to other processes, and the algorithm can adapt accordingly to prevent deadlock.
Q8: Are there real-world applications of the Banker’s Algorithm?
A: Yes, the Banker’s Algorithm has real-world applications in various fields, including computer systems, finance, and manufacturing. For example, it is used in resource management in operating systems, ensuring efficient utilization of resources while preventing system-wide deadlocks.
Q9: What is the worst-case time complexity of the Banker’s Algorithm?
A: The worst-case time complexity of the Banker’s Algorithm is O(m * n^2), where ‘m’ is the number of resource types and ‘n’ is the number of processes. This is because, in the worst case, the algorithm may need to check all resource allocation possibilities.
Q10: Are there variations of the Banker’s Algorithm for different scenarios?
A: Yes, there are variations and extensions of the Banker’s Algorithm to accommodate different scenarios, such as multiple resource instances, priority-based allocation, and more complex resource allocation policies. These variations address specific requirements in diverse environments.
Conclusion
The Banker’s Algorithm is a powerful tool for managing resources and preventing deadlocks in operating systems. Understanding its core concepts and implementing it in C can be a valuable skill for systems programmers and developers. In the next part of this series, we will delve into the code to complete the implementation and check for safe states. Stay tuned!